In
this article I am going to explain how to insert record into Sql server
database using Store Procedure and Entity framework in asp.net
Description:
In
the previous article I have explained How to insert record into Sql server databaseusing Entity framework in asp.net, Howto set up ADO.net Entity Framework project or website in asp.net, Search records from Sql Server database using Linq to Sql and How to show confirmation alert before closing browser tab using JavaScript.
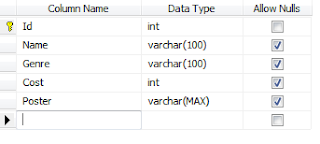
Create
a Store procedure to insert data:
Create PROCEDURE
Sp_InsertMovieDetail
(
@name varchar(100),
@genre varchar(100),
@cost int,
@poster varchar(max)
)
AS
BEGIN
SET
NOCOUNT ON;
Insert into Movie(Name,Genre,Cost,Poster) values(@name,@genre,@cost,@poster)
END
To
set up the ADO.net entity framework project read the article How to set upADO.net Entity Framework project or website in asp.net.
After
that add a webform to project/website.
HTML Markup
of webform:
<fieldset
style="width:450px;">
<legend>Entity Framework Tutorial</legend>
<table>
<tr>
<td>Movie Name :</td>
<td><asp:TextBox ID="txtname" runat="server" /></td>
</tr>
<tr>
<td>Genre :</td>
<td><asp:TextBox ID="txtgenre" runat="server" /></td>
</tr>
<tr>
<td>Cost :</td>
<td><asp:TextBox ID="txtcost" runat="server" /></td>
</tr>
<tr>
<td>Upload Movie banner :</td>
<td>
<asp:FileUpload ID="fubanner" runat="server" /></td>
</tr>
<tr><td></td><td>
<asp:Button ID="Button1" runat="server" Text="Submit" OnClick="Button1_Click" CausesValidation="false" /></td></tr>
</table>
</fieldset>
Create the
object of Entity connection
C#:
DemoEntities db = new DemoEntities();
VB:
Dim db As New DemoEntities
On
button click write the code to insert the record and clear the control.
C#:
protected void Button1_Click(object sender, EventArgs e)
{
try
{
string
filepath = Server.MapPath("~/images/")
+ Guid.NewGuid() +
fubanner.PostedFile.FileName;
fubanner.SaveAs(filepath);
string
fl = filepath.Substring(filepath.LastIndexOf("\\"));
string[]
split = fl.Split('\\');
string
newpath = split[1];
string
imagepath = "~/images/" + newpath;
db.Sp_InsertMovieDetail(txtname.Text, txtgenre.Text, Convert.ToInt32(txtcost.Text),
imagepath);
Response.Write("<script>alert('Record
Insert Successfully');</script>");
Clear();
}
catch(Exception ex)
{ }
}
public void Clear()
{
txtname.Text = string.Empty;
txtcost.Text = string.Empty;
txtgenre.Text = string.Empty;
}
VB:
Protected Sub
Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
Try
Dim filepath As String = Server.MapPath("~/images/") & Guid.NewGuid().ToString() &
fubanner.PostedFile.FileName
fubanner.SaveAs(filepath)
Dim fl As String = filepath.Substring(filepath.LastIndexOf("\"))
Dim split As String() = fl.Split("\"c)
Dim newpath As String = split(1)
Dim imagepath As String = Convert.ToString("~/images/") & newpath
db.Sp_InsertMovieDetail(txtname.Text,
txtgenre.Text, Convert.ToInt32(txtcost.Text),
imagepath)
Response.Write("<script>alert('Record
Insert Successfully');</script>")
Clear()
Catch ex As Exception
End Try
End Sub
Public Sub Clear()
txtname.Text = String.Empty
txtcost.Text = String.Empty
txtgenre.Text = String.Empty
End Sub
Build
the project and run. Test the application via insert the record.
Demo:
In this article we have learn to how to insert record into sql server database using Store Procedure and Entity Framework 6.0 in asp.net Visual studio 2013. I hope you enjoyed this article.
No comments:
Post a Comment